Get content from your project
Getting content from your project is one of the core responsibilities of your app.Discover how to use basic filtering to retrieve some of your project’s published content items, such as articles.
Which API to use?
While your content creators write articles and add finishing touches to the content in your project, you can display that content in web and mobile apps using Delivery API. Delivery API is a read-only API that’s available as both REST API and GraphQL API. Here you’ll learn the basics of using the Delivery REST API for getting published content.Make a request
To retrieve content items from a project, you need to specify the project’s environment ID. You can find your environment ID in the environment settings. Let’s see how to get all content items from the specified environment.// Tip: Find more about JS/TS SDKs at https://kontent.ai/learn/javascript
import { createDeliveryClient } from '@kontent-ai/delivery-sdk';
const deliveryClient = createDeliveryClient({
environmentId: '8d20758c-d74c-4f59-ae04-ee928c0816b7'
});
const response = await deliveryClient.items()
.toPromise();
Filter items by type
You know how to retrieve all items. Let’s expand by retrieving items based on a specific type. In this example, you’ll retrieve items based on the Article content type.1. Find the codename
Before moving further, you need to find the codename of the content type.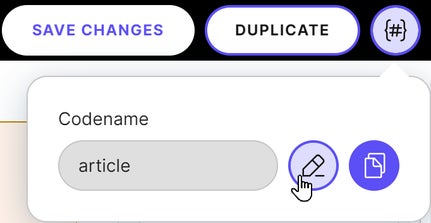
2. Filter by type’s codename
The information about a content item’s type is stored in the content item’s System object property. The System object contains metadata about the content item, such as the last content modification date, language, content type, and more.{
"system": {
"id": "335d17ac-b6ba-4c6a-ae31-23c1193215cb",
"collection": "default",
"name": "My article",
"codename": "my_article",
"language": "en-US",
"type": "article",
"sitemap_locations": [],
"last_modified": "2023-03-27T13:21:11.38Z",
"workflow_step": "published"
},
"elements": {
...
}
}
type
property. Any content items not based on the Article content type will be omitted from the API response.
// Tip: Find more about JS/TS SDKs at https://kontent.ai/learn/javascript
import { createDeliveryClient } from '@kontent-ai/delivery-sdk';
import { Article } from './models/Article';
const deliveryClient = createDeliveryClient({
environmentId: '8d20758c-d74c-4f59-ae04-ee928c0816b7',
});
const response = await deliveryClient.items<Article>()
.type('article')
.toPromise();
Order the items
By default, the Delivery API sorts content items alphabetically by their codenames. With content like articles, you might want to use chronological order. For example, have the articles ordered by their last modified date.To retrieve content items in a specific order, specify the following:
- The content item property to order by. For example, the property can be a content element (such as text or date & time element) or content item metadata (such as the item’s type or last modified date).
- Whether to use the ascending or descending order.