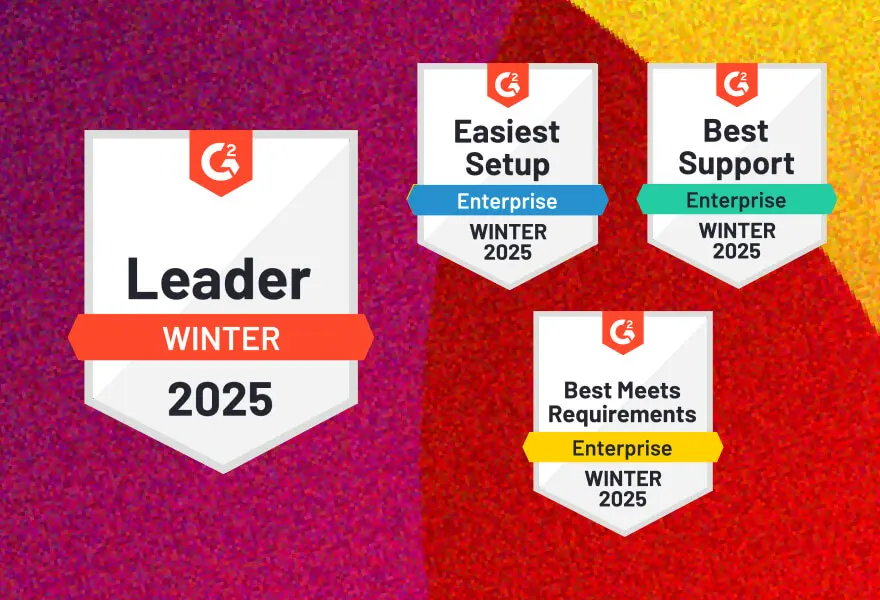
Kontent.ai named Winter Leader on G2 with badges in Enterprise CMS categories
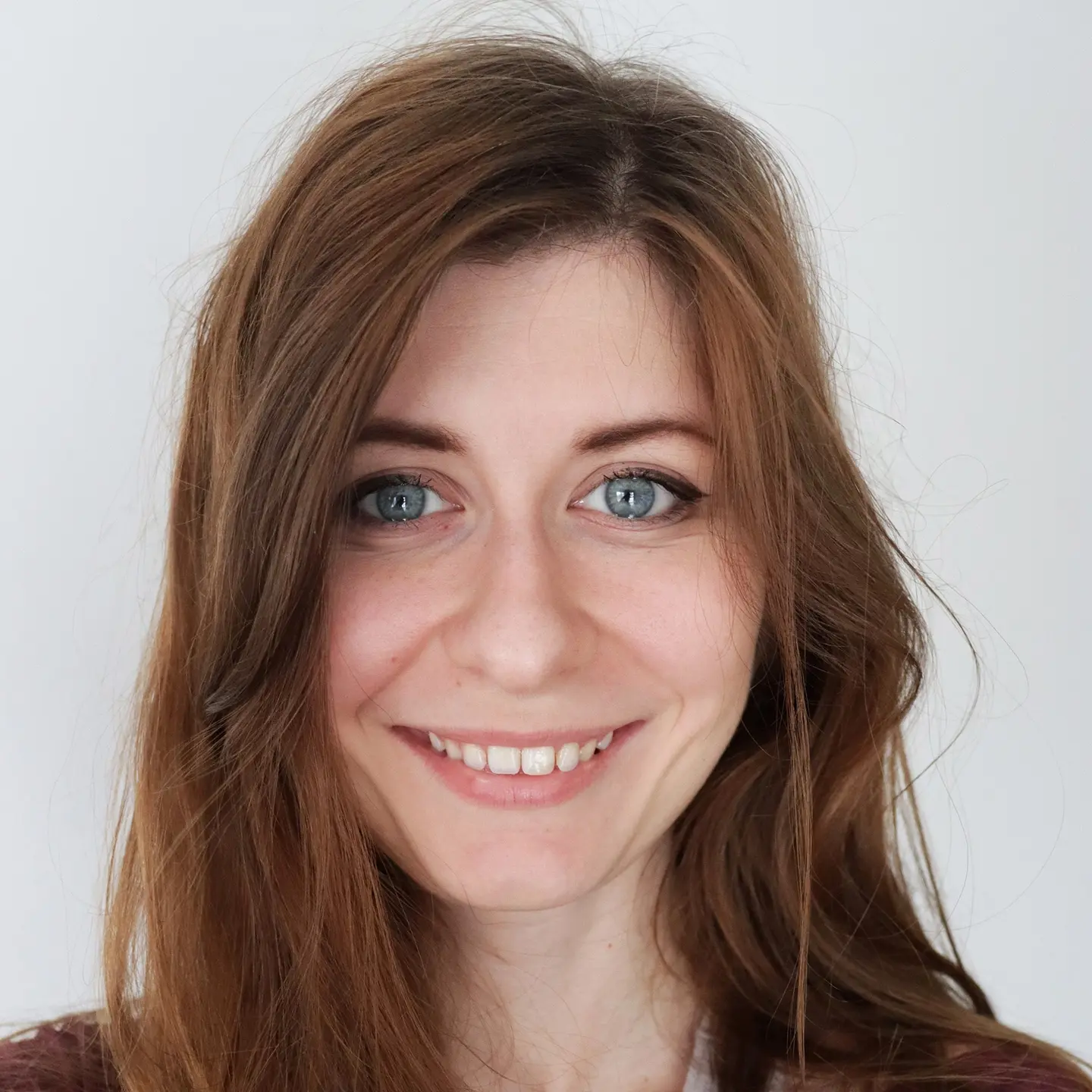
Zaneta Styblova
Jan 8, 2025
Zaneta Styblova
Jan 8, 2025
Lucie Simonova
Sep 30, 2024
Monica Raszyk
Dec 16, 2024
Learn how to generate unparalleled return on your content with Kontent.ai.