Headless, Python, and Flask: How to enjoy massive flexibility in a microframework
Project requirements evolve and successful websites change over time. How can we manage website growth with Python?
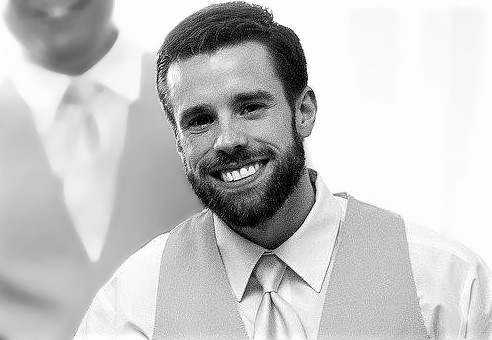
Published on Jun 3, 2021
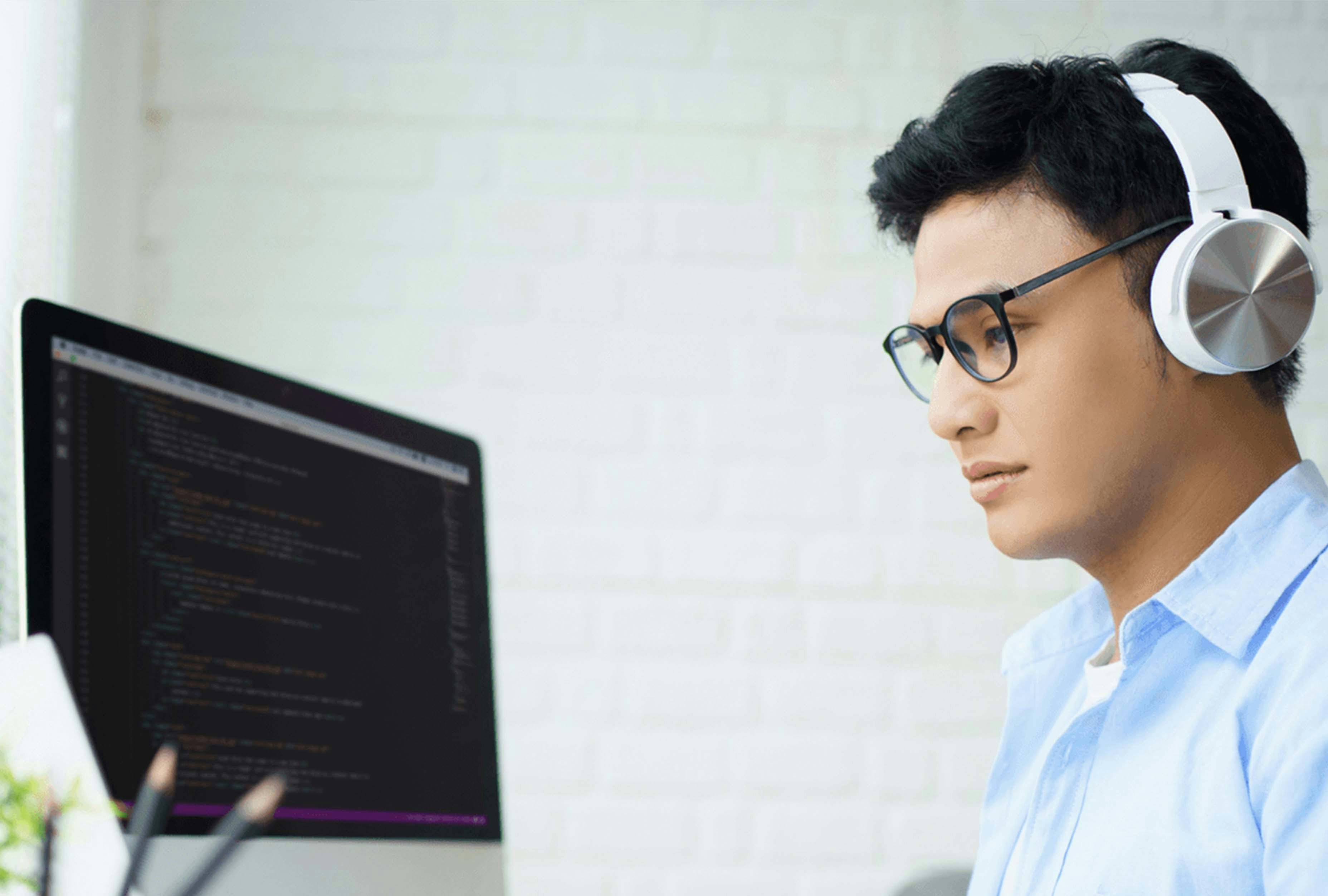
Project requirements evolve and successful websites change over time. How can we manage website growth with Python?
Published on Jun 3, 2021
In this article, I’ll show you how to feed Flask application data from a headless CMS and discuss why pairing the two results in a more flexible website architecture. If you’re new to Flask, take a look at the user’s guide in the official Flask documentation. It has a wonderful foreword, quick-start introduction, and full-length tutorial.
Weird opener, I know, but bear with me. Snakes grow until they die. Their growth slows down a lot as they mature, but it doesn’t stop if they are being fed. I’d like to think I can apply this to my own life and skills. Learn something everyday, practice your skills frequently, keep advancing and refining whatever you’re working on, feed yourself new information.
I enjoy writing code in Python. It’s explicit, it has a low entry-learning curve, it’s human readable, and it has a wide variety of uses. Python projects range from simple file system CRUD scripts to robust machine learning libraries. With Python, I feel as I grow in skill, it always has a place for me.
Using Python has become a “labor of love” for me, and I wanted to extend that same welcoming feeling to the web development projects I regularly work on. I needed a web framework that starts small but can scale in complexity as a project advances. Flask fits the bill perfectly.
Flask is a minimal Python web development framework that bridges your application code to a Web Server Gateway Interface (WSGI) and templates. It doesn’t come with a database layer, form layer, or any other features. The official documentation says it best:
“Flask can be everything you need and nothing you don’t...As your codebase grows, you are free to make the design decisions appropriate for your project. Flask will continue to provide a very simple glue layer to the best that Python has to offer.”
If your application was a meal, Flask isn’t even the “meat and potatoes,” it’s the plate and the fork.
Flask’s flexibility is hard to summarize succinctly because of all the extensibility options it has, but in the interest of brevity, I’ve focused on scalability and ease of development:
For example, in the diagram, you can see three different types of client requests that your site may service in the future:
If you only need to serve up simple pages, then you may only need your Flask application on a server. However, as time passes, you might find that you need to add “login” support with extensions, a media server with middleware, or blueprints for dynamic routing. Flask can scale to meet these scenarios.
@app.route('/')
def index():
return 'Index Page Text'
@app.route('/hello')
def hello():
return 'Hello, World!'
# in file: home/view.py:
return render_template("home/index.html", hero=hero, articles=articles)
# in file: templates/home/index.html
<h2>{{ hero.elements.title.value }}</h2>
There’s a lot to consider when it comes to selecting a framework and CMS, but cost, complexity, and future-proof design are always tied to whether a project is flexible. Here’s why using a headless CMS and Flask meet these requirements head-on:
Designing your project using a microservices architecture translates to a smaller code footprint. Using a microframework means there isn’t any “solution bloat” with features. You’ll use only the best-of-breed integrations, and you can incrementally add those tools as your project matures.
Similar to the scalability diagram earlier, here’s what integrating with external services may look like to handle different client scenarios:
Using a SaaS headless CMS means your management system’s code is hosted by the vendor, resulting in zero overhead for you. Additionally, the content stays isolated from the project’s code, and you typically don’t need code changes when new CMS features are released.
Headless CMSs provide content in an omnichannel capable format, meaning the content is reusable across different types of applications throughout your organization. With Python being a prime language for machine learning, AI, and data engineering, this could prove extremely useful for the future of your content!
With a headless CMS subscription payment model, you only pay for what you need and can typically adjust the subscription plan to fit your budget and requirements.
Flask is open-source and free. I’d say that’s the most flexible charge your company credit card has ever seen.
Warmups and exercise are the best way to maintain flexibility, so in this section, let’s run through a sample site setup to see Flask and a headless CMS in action.
We’ll use Kontent.ai as our headless CMS, a Kontent.ai Python SDK, the larger application structure advised by Flask, and a shared Kontent.ai project.
Full disclosure: I built this “unofficial Kontent Python SDK” and connecting Flask to Kontent.ai can be done with just an HTTP request library. However, it did save me a lot of code in my views:
We’ll set up the site in five steps:
py -m venv env
pip install flask
pip install kontent_delivery
sample/
__init__.py
home/
views.py
templates/
base.html
home/
index.html
config.py
setup.py
from setuptools import setup
setup(
name='kontent_flask',
packages=['sample'],
include_package_data=True,
install_requires=[
'flask',
],
)
project_id ="975bf280-fd91-488c-994c-2f04416e5ee3"
delivery_options = {
"preview": False,
"preview_api_key": "enter_key_here",
"secured": False,
"secured_api_key": "enter_key_here",
"timeout": (4,7)
}
from flask import Flask
import config
from kontent_delivery.client import DeliveryClient
app = Flask(__name__)
client = DeliveryClient(config.project_id, options=config.delivery_options)
import sample.home.views
from flask.templating import render_template
from sample import app, client
@app.route("/")
def index():
resp = client.get_content_item("home")
if resp:
hero_units = resp.get_linked_items("hero_unit")
hero = hero_units[0]
articles = resp.get_linked_items("articles")
return render_template("home/index.html", hero=hero, articles=articles)
# custom 404 page information available at:
# https://flask.palletsprojects.com/en/2.0.x/errorhandling/?highlight=404#custom-error-pages
return render_template("error_pages/404.html"), 404
<html>
<head id="head">
{% block head %}
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta charset="UTF-8" />
<title>Flask Sample</title>
{% endblock %}
</head>
<body>
<div class="container">
{% block content %}
{% endblock %}
</div>
</body>
</html>
{% extends "base.html" %}
{% block content %}
<h1>Flask Simple Example</h1>
<h2>{{ hero.elements.title.value }}</h2>
<div>
{% for article in articles %}
<div>
<div>
<h2>
<a href="/articles/{{article.elements.url_pattern.value}}">
{{ article.elements.title.value }}
</a>
</h2>
<p>
{{ article.elements.summary.value }}
</p>
<div>
{{ article.elements.post_date.value }}
</div>
</div>
</div>
{% endfor %}
</div>
{% endblock %}
set FLASK_APP=sample
pip install -e .
(including the period)flask run
Following the Flask application link from the terminal (http://127.0.0.1:5000 by default) will bring you to your Kontent + Flask site. If you have a Kontent account and want to test this out with your own sample Kontent project, follow these instructions.
The full code for this sample can be seen in this GitHub repository.
In this article, we discussed the benefits of using Flask with a headless CMS and how to create a Flask + Kontent.ai sample project. Now I’m sure you’ll agree that using a microframework with a headless CMS affords major flexibility!
If this article got you excited about the possibilities of using Flask and Kontent.ai, a more robust Flask + Kontent.ai sample site can be seen here.
Are you having issues with the Flask samples from the article? Get in touch on our Discord.