What does native GraphQL support in Kontent.ai bring for devs?
GraphQL or REST API? What is the best way to fetch content from a headless CMS, and are there any disadvantages?
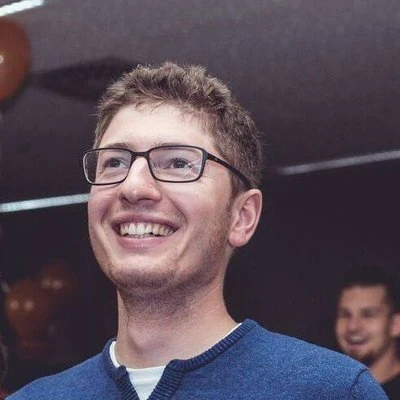
Published on Nov 29, 2021
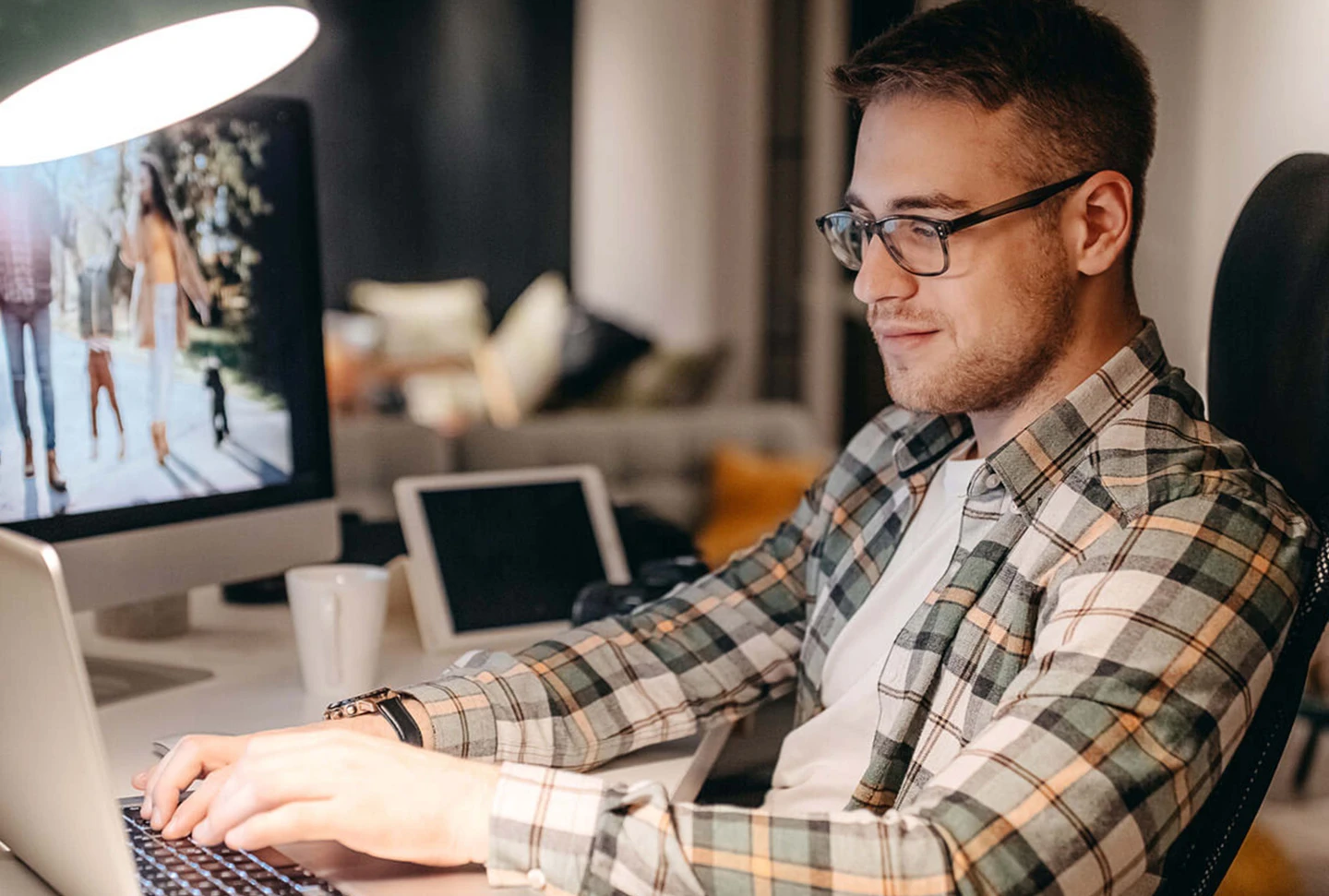
GraphQL or REST API? What is the best way to fetch content from a headless CMS, and are there any disadvantages?
Published on Nov 29, 2021
In this article, I will introduce Kontent.ai’s new native GraphQL endpoint, explain GraphQL benefits from the developer’s point of view, show how to use it in JavaScript, and compare GraphQL to REST API to find out if and how one is significantly better than the other.
GraphQL is a way of fetching data. It’s an alternative to REST API, and many call it the “new” way of getting data. These are my top reasons for using GraphQL:
This is what a simple GraphQL query looks like:
query {
content_All (limit: 3){
items {
title,
teaser {
html
},
localSlug
}
}
}
Note: content
in this context is a codename of a content type.
It fetches titles, teasers, and slugs of 3 articles for a website homepage, as that’s all we need for that page. The response is minimalistic JSON:
{
"data": {
"content_All": {
"items": [
{
"title": "Flexbox vs Grid - How...",
"teaser": {
"html": "<p>There are so many..."
},
"localSlug": "flexbox-vs-grid"
},
{
"title": "Can You Switch Your Headless...",
},
{
"title": "Gatsby source plugin...",
}
]
}
}
}
So, where are we on the “no need to read docs” point? How do we actually know that there are articles and that they each have a title and a teaser?
GraphQL comes with a schema. It’s a map of all your content, and it’s a great source of information for GraphiQL or GraphQL Playground (works online) that allows you to build and test your queries. Let’s take a look at it.
Open the online playground. First, you need to add the Kontent.ai endpoint URL, which is https://graphql.kontent.ai/<YOUR-PROJECT-ID>:
When you enter the playground, expand the Schema tab on the right. You’ll see the generated schema of your project:
But that’s not the best part. On the left, write this query syntax:
query {
}
And hit CTRL+SPACE (or OPTION+SPACE on Mac) with your cursor within the curly brackets. You’ll see an IntelliSense popup that shows you all available data:
We don’t really need to know the complete project structure or understand the API specifics. We can navigate through the available items and mark the data and properties we need for our use case. Curly brackets expand the current item, parentheses let you filter, limit, and order. The play button executes the query and shows you the fetched data:
Try it out with your project and build a query that’s useful for your project.
Now that we have our query, how can we use it in our JS projects?
With REST API, we were used to leveraging third-party tools like Axios, Request, or Fetch (which is now widely available in browsers) to compose and execute the queries. With GraphQL, we need to use Apollo Client:
npm install @apollo/client graphql
We create the client and configure it to use the right endpoint:
import { ApolloClient, InMemoryCache } from '@apollo/client'
...
const client = new ApolloClient({
cache: new InMemoryCache(),
uri: 'https://graphql.kontent.ai/<YOUR-PROJECT-ID>'/
})
Note: If you’re using React and need the client on many pages throughout your app, you can use the ApolloProvider
component that wraps your React app and lets you use React hook useQuery
to fetch data.
Then, you can start fetching data with your GraphQL queries:
import { gql, useQuery } from '@apollo/client'
...
const response = await client.query({
query: gql`
query {
content_All (limit: 3){
items {
title,
teaser {
html
},
localSlug
}
}
}
})
console.log(response)
The client returns data in JSON, so you can directly use it in your components:
response.data.content_All.items.map((article) =>
<Article data={article} />
)
So what’s better, GraphQL or REST API? Let’s do a quick pros and cons list:
GraphQL | REST API | |
Pros | The syntax of queries is unified, so if you hire a new developer, they don’t need to understand the API prior to working with its data. The data fetching, filtering, ordering, and other operations have the same syntax among various data providers. | It is a very robust, universal, and proven way of data communication. |
Cons | If your GraphQL queries are too complex, their syntax becomes unreadable even if you have an idea of “what’s going on.” | Almost all REST queries suffer from overfetching, underfetching, or both, even though APIs provide ways to resolve or minimize this. That affects performance, used bandwidth, etc. |
I’m afraid there’s no clear winner. As always, it depends on your project and its requirements.
GraphQL was originally created to streamline data fetching from multiple sources—to allow developers to focus on their implementation rather than spend half of their days reading docs. And it does that perfectly. GraphQL can make a real difference in data fetching simplicity for large projects that require content from Kontent.ai, products from Shopify, user data from the PostgreSQL database, and so on. If you’re interested in this topic, you can check out my article about Apollo.
For smaller projects where we mainly work with content from a single source—the headless CMS—GraphQL is nothing new. We’ve already seen it in Gatsby or Gridsome, and we’ve learned that it helps with development speed a lot. If you manage to keep your queries at a reasonable size, there are not many disadvantages. Especially now when Kontent.ai features a native GraphQL endpoint hosted on the global Fastly CDN.
In this article, I explained what GraphQL is and how it compares to REST API. I showed you how to build GraphQL queries using the visual builder connected to your project’s endpoint and how to fetch content with GraphQL in JavaScript projects.
If you’re interested in GraphQL and React, definitely check out the Kontent.ai React GraphQL sample app on our GitHub. And if you have any questions or comments, join our Discord and let us know!
What if we told you there was a way to make your website a place that will always be relevant, no matter the season or the year? Two words—evergreen content. What does evergreen mean in marketing, and how do you make evergreen content? Let’s dive into it.
Lucie Simonova
How can you create a cohesive experience for customers no matter what channel they’re on or what device they’re using? The answer is going omnichannel.
Zaneta Styblova
In today’s world of content, writing like Shakespeare is not enough. The truth is, there are tons of exceptional writers out there. So what will make you stand out from the sea of articles posted every day? A proper blog post structure.
Lucie Simonova