How to generate RSS feed in Next.js
It seems the RSS era is long gone, but there are still many valid use cases for generating RSS feeds. If your Next.js site has lots of content, how can you generate an RSS feed?
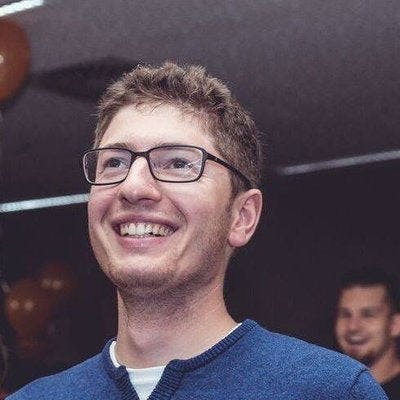
Published on Oct 10, 2022

It seems the RSS era is long gone, but there are still many valid use cases for generating RSS feeds. If your Next.js site has lots of content, how can you generate an RSS feed?
Published on Oct 10, 2022
In this article, I will explain what RSS is and how it is typically used. I will introduce the NPM package rss
and share code samples of how it can be integrated into an existing Next.js site powered by the modular content platform Kontent.ai.
RSS stands for Really Simple Syndication and it allows you to distribute up-to-date content from your website to other websites, RSS readers, and other channels. Essentially, it’s just structured content in an XML format:
<?xml version="1.0" encoding="UTF-8" ?>
<rss version="2.0">
<channel>
<title>Kontent.ai</title>
<link>https://kontent.ai/</link>
<description>Kontent.ai is a modular content platform that offers easy-to-use content management tools for marketers while freeing developers to focus on building online experiences.</description>
<item>
<title>Kontent.ai has raised $40 million in growth capital</title>
<link>https://kontent.ai/blog/kontent-ai-has-raised-40-million-in-growth-capital/</link>
<description>Today, we’re excited to introduce ourselves as Kontent.ai and share some big investment news! Learn more about the new company and our vision for the future.</description>
</item>
<item>
...
</item>
...
</channel>
</rss>
In the past, people used to have RSS readers on their devices, which would allow them to monitor multiple sources of content easily. Nowadays, RSS feeds are more often used for integrations between websites, services, or applications.
For example, we use our own RSS feed to notify internal teams about published blog posts:
Another example is Zapier, the process automation platform, which includes RSS as one of its most common triggers:
The types of sites that can benefit from RSS the most are the content-heavy ones. News sites, blog sites, but also pages showing changelogs, releases, and similar.
To generate an RSS feed, we first need to decide what content we want to include. It doesn’t make sense to build an XML version of the whole website, but only add the type of content I outlined above. In the case of our website, that means blog posts and customer success stories:
const blogPostsData = await client
.items<BlogPostModel>()
.type(contentTypes.blog_post.codename)
.orderByDescending(getElementsParamCodename(contentTypes.blog_post.elements.date.codename))
.toPromise()
const caseStudiesData = await client
.items<CaseStudyModel>()
.type(contentTypes.case_study.codename)
.orderByDescending(getElementsParamCodename(contentTypes.case_study.elements.publish_date.codename))
.toPromise()
Note: The RSS package docs page says: “Most feeds use 20 or fewer items.” Based on this recommendation, you may limit the number of returned items from the API.
But where do we put the code?
We have two options:
For a long time, we used the first option and added the RSS generation code to index.tsx
page. This approach is fine as long as you’re happy with having an extra piece of code in the page implementation, and the page is always treated as a static page. We weren’t happy with that as it makes the code hard to find, increases maintenance, and complicates the onboarding of new developers. We wanted to keep only page-related code in the page implementation. So we switched to using a pre-build script.
I explained the pre-build scripts and the mechanism to execute them before every build in my other article, so here I’ll just show you the actual script code.
First of all, we need to install the RSS package:
npm i rss --save
The package will do the heavy lifting for us, we just need to provide the data in the RSS structure. We’ll start by defining the feed basics:
// create a new feed object
const feed = new RSS({
title: 'Our great RSS feed',
description: 'All our blog posts and customer success stories news in one place.'
feed_url: 'https://oursite.com/rss.xml',
site_url: 'https://oursite.com',
copyright: '(c) 2022 Our company',
})
Now, to add the content to the feed, we need to provide the following fields:
In our case, we need to process multiple content types, so for the sake of this example, I simplified the code to a single content type:
blogPostsData.forEach(blogPost => {
feed.item({
title: blogPost.elements.title.value,
description: blogPost.elements.perex.value,
author: blogPost.elements.author.linkedItems[0]?.elements.name.value,
date: new Date(blogPost.elements.date.value),
URL: `${getUrlByContentType(contentTypes.blog_post.codename, post.elements.urlSlug.value)}?utm_source=rss&utm_medium=feed`,
})
})
The final step is to save the file in the desired location. In the code above, it was defined as /public/rss.xml
. The public folder in Next.js is used to store static assets like images and downloadable files.
const fullFilePath = path.join(process.cwd(), 'public', 'rss.xml')
// remove the old file
if (fs.existsSync(fullFilePath)) {
await fs.promises.unlink(fullFilePath)
}
fs.writeFile(fullFilePath, feed.xml(), err => {
if (err) {
console.log('Error: ', err)
}
console.log('RSS feed generation: all good')
}
Note: Don’t forget to add the /public/rss.xml
to your .gitignore
file so that you don’t track this dynamic file.
Depending on your desired use of the RSS feed, you can add it to your site with a simple link:
<Link href="/rss.xml" passHref>
<a>RSS</a>
</Link>
Or a header meta tag:
<link rel="alternate" type="application/rss+xml" title="Our great RSS feed" href="/rss.xml" />
In this article, I explained what RSS is, outlined some of the use cases for RSS feeds like integrations, and showed you how to generate an RSS feed using the RSS package on a Next.js website either via a statically generated page or pre-build script. In the end, I provided two examples of linking to the RSS feed using a simple link or a header meta tag.
If you’re building a Next.js site and are looking for more related posts, make sure to join our Discord so you don’t miss any new discussions and articles.