Secure access
Protect your content and assets with secure access. You might want to enable secure access with sensitive content, content hidden behind sign-in walls, or for projects that are not public facing.Without secure access, your assets and published content items are publicly available by default.
Enable secure access
When you activate secure access, Delivery API requires an API key with each API request for content. This applies to both Delivery REST API and Delivery GraphQL API.- In Kontent.ai, go to
Environment settings > General.
- In Enabled APIs, use the toggle to activate Secure access for Delivery API.
- In
Project settings > API keys, create a Delivery API key with permission for secure access.
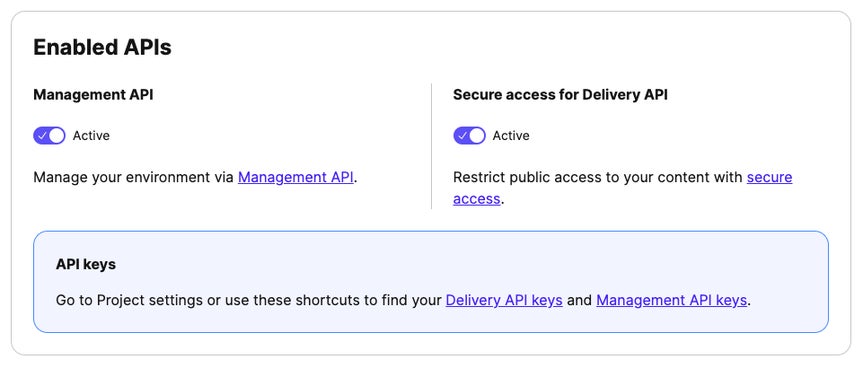
Retrieve content items securely
- Retrieve content on the server side and NOT on the client side to prevent leaking your API keys.
- Store your API Keys outside your source code. For example, store them as environment variables. Make sure they’re encrypted too.
- Rotate your API keys periodically. The older an API key is, the higher the probability it could have been compromised.
- Before you regenerate or revoke an API key, ensure your apps use a new API key to prevent downtime.
// Tip: Find more about JS/TS SDKs at https://kontent.ai/learn/javascript
import { IContentItem, createDeliveryClient, Elements } from '@kontent-ai/delivery-sdk';
// Tip: Create strongly typed models according to https://kontent.ai/learn/strongly-typed-models
export type Article = IContentItem<{
title: Elements.TextElement;
summary: Elements.TextElement;
post_date: Elements.DateTimeElement;
teaser_image: Elements.AssetsElement;
related_articles: Elements.LinkedItemsElement<Article[]>;
}>;
const deliveryClient = createDeliveryClient({
environmentId: 'KONTENT_AI_ENVIRONMENT_ID',
defaultQueryConfig: {
useSecuredMode: true // Queries the Delivery API using secure access.
},
secureApiKey: 'KONTENT_AI_DELIVERY_API_KEY',
});
const response = await deliveryClient
.item<Article>('my_article')
.toPromise();
Retrieve assets securely
Do you manage confidential assets or need to run an intranet website? In that case, you might want to keep your assets away from the public. You can restrict access to your assets by requiring an API key. This API key differs from the API keys in- Your environment ID
- Whether to enable secure assets for the Delivery Preview API, Delivery API, or both
This feature isn’t available for some legacy plan subscriptions. Contact us to find out your options.